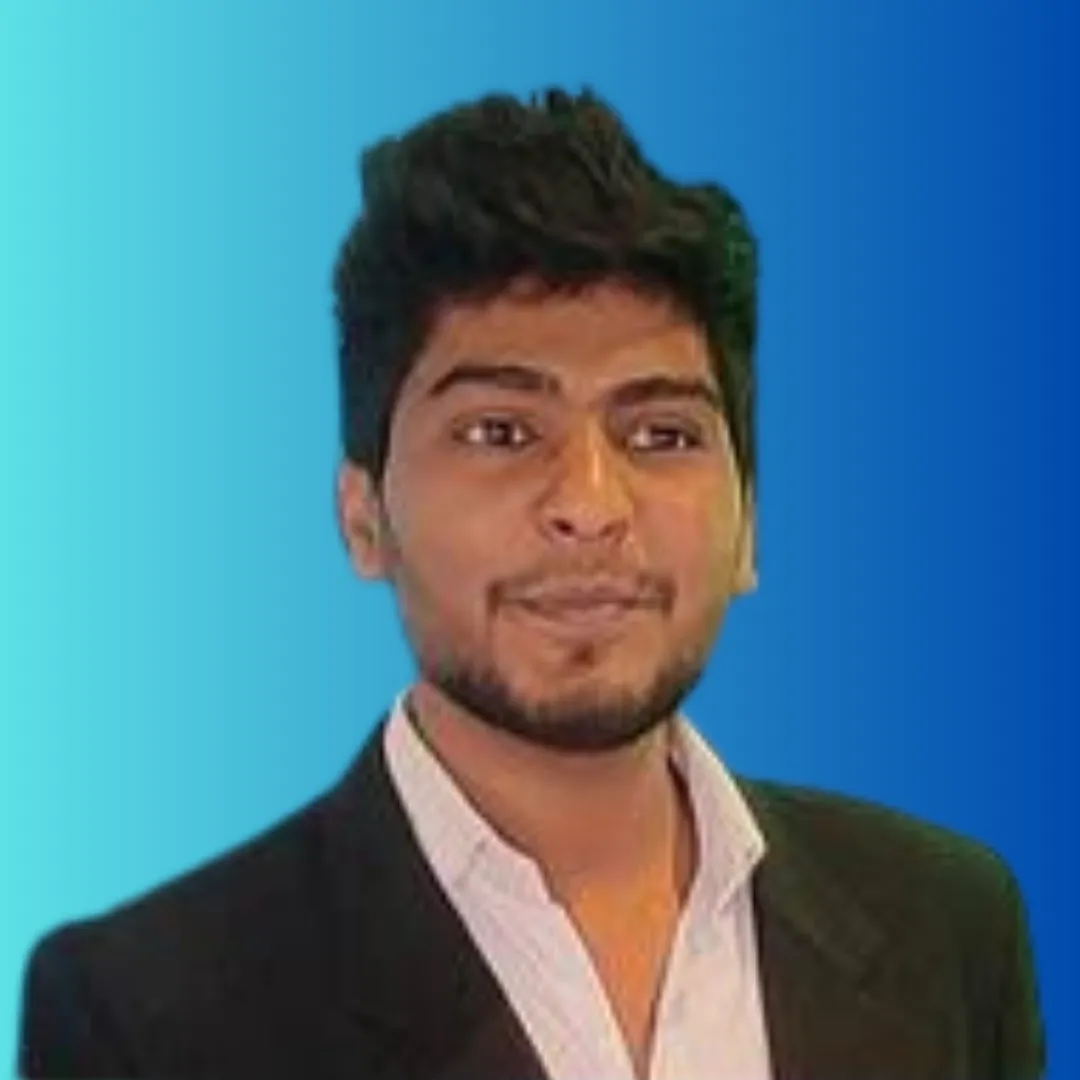
Nitish Goyal
Senior Android Engineer
Introduction
In the ever-evolving world of Android development, staying ahead requires embracing the latest tools and frameworks that streamline your workflow while enhancing the user experience. One such groundbreaking tool is Jetpack Compose, a modern UI toolkit that has redefined how you build Android applications. This comprehensive guide is designed to help you master Jetpack Compose and harness its full potential in your app development journey.
Overview of Jetpack Compose
Jetpack Compose is Google's cutting-edge UI toolkit that allows you to build native Android UIs with a declarative approach. Unlike the traditional XML-based UI development, Jetpack Compose is entirely Kotlin-based, making your code more intuitive, concise, and easier to maintain. It enables you to define your UI components directly in the Kotlin code, eliminating the need for complex XML layouts and bridging the gap between the UI and logic.
Jetpack Compose integrates seamlessly with existing Android applications, making it a powerful tool for both new projects and those looking to modernize their UI without a complete overhaul. Its modular and flexible design empowers you to create complex UIs effortlessly while maintaining high performance and responsiveness.
Why Did Google Introduce Jetpack Compose?
Jetpack Compose was introduced to address the complexities and limitations of the existing View-based UI framework. Here’s why Google felt the need for it:
- XML Complexity: Android’s previous UI system required separating UI definitions (XML) from logic (Kotlin/Java), increasing the complexity of UI development.
- Boilerplate Code: Managing UI state and ensuring it was in sync with the backend often resulted in excessive boilerplate code.
- Performance Bottlenecks: As UI complexity grew, the traditional View system struggled with performance, especially with deeply nested layouts.
Jetpack Compose simplifies these issues by offering a declarative UI framework:
- Unified Codebase: Instead of managing XML and Kotlin separately, UI and logic are written together in Kotlin.
- State-Driven: With Compose’s declarative nature, you describe the UI as a function of its state. This makes UI changes automatic when the underlying state changes.
- Kotlin-Powered: Compose is built with Kotlin, utilizing features like coroutines, lambdas, and extension functions to make development faster and more intuitive.
Advantages Over Traditional UI Development
Jetpack Compose offers significant advantages over traditional XML-based UI development, particularly in efficiency, maintainability, and developer experience:
- Declarative Approach: Compose uses a declarative style, where the UI is defined based on the app's current state, automatically updating as the state changes. This reduces manual UI management, minimizes bugs, and simplifies the code. In contrast, XML layouts follow an imperative style, leading to more complex code when handling dynamic content.
- Kotlin-Based: Leveraging Kotlin's features like type safety and extension functions, Jetpack Compose ensures a more robust codebase with fewer runtime errors. It reduces boilerplate, as data-binding and view references are encapsulated within composable functions, streamlining development.
- Less Boilerplate: Compose eliminates the need for repetitive code, such as view bindings and XML definitions, by using reusable composable functions. This speeds up development and enhances code readability and maintainability.
- Seamless UI State Management: Compose simplifies state management with reactive tools like State and LiveData. The UI automatically updates in response to state changes, ensuring synchronization with underlying data, unlike the manual state management required in XML-based UI.
How Jetpack Compose Works Internally
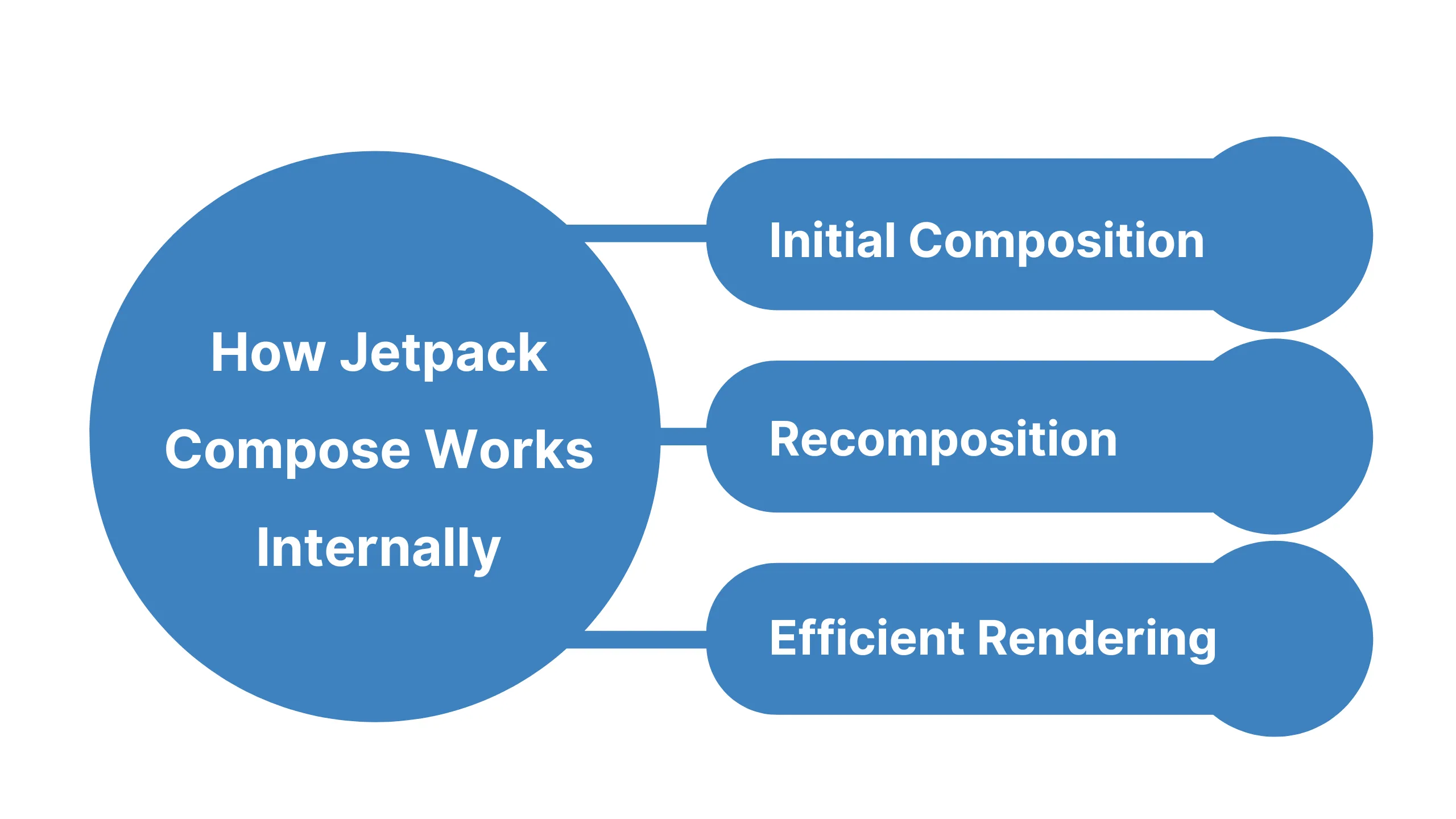
Jetpack Compose functions by managing UI components declaratively, leveraging Kotlin’s powerful capabilities to create reusable composable functions. Here's a high-level view of how it works:
Environment Setup
To harness the full potential of Jetpack Compose, it's crucial to have the right development environment configured. Here's a step-by-step guide to ensure you're set up for success.
- Required Android Studio Version: Jetpack Compose is tightly integrated with Android Studio, so you must use a version that fully supports Compose. As of now, ensure you have Android Studio Giraffe | 2022.3.1 or higher. This version comes with enhanced support for Jetpack Compose, including tools for previewing UI components and testing Compose code.
- Dependencies and Gradle Configurations: In order to use Jetpack Compose, you need to configure your project’s build.gradle files correctly. Start by ensuring that your project is using the Kotlin 1.8.0 or later, as Jetpack Compose relies heavily on Kotlin's modern features.
Project-level build.gradle
file:
buildscript {
ext.kotlin_version = "1.8.0"
dependencies {
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
classpath "com.android.tools.build:gradle:8.0.0"
}
}
plugins {
id 'com.android.application'
id 'kotlin-android'
}
android {
compileSdkVersion 34
defaultConfig {
applicationId "com.example.yourapp"
minSdkVersion 21
targetSdkVersion 34
versionCode 1
versionName "1.0"
}
buildFeatures {
compose true
}
composeOptions {
kotlinCompilerExtensionVersion '1.4.8'
}
kotlinOptions {
jvmTarget = '1.8'
}
}
dependencies {
implementation "androidx.compose.ui:ui:1.4.8"
implementation "androidx.compose.material:material:1.4.8"
implementation "androidx.compose.ui:ui-tooling-preview:1.4.8"
implementation "androidx.lifecycle:lifecycle-runtime-ktx:2.6.1"
implementation "androidx.activity:activity-compose:1.7.2"
}
Kotlin Language Features Required
Jetpack Compose is built to take full advantage of Kotlin's modern language features. To master Compose, you should be proficient with:
- Coroutines: Handle asynchronous tasks effectively, especially when dealing with UI events, data loading, or background processing. Jetpack Compose integrates seamlessly with Kotlin Coroutines, allowing for smooth, responsive UI interactions.
- Flow: This is a powerful component for handling streams of data asynchronously, making it essential for managing state in your Compose applications.
- Kotlin DSL: Compose uses a declarative UI approach, which is heavily reliant on Kotlin’s domain-specific language (DSL) capabilities. Familiarize yourself with Kotlin’s DSL syntax to write clean, readable Compose code.
Project Configuration
Now that you’ve set up your environment, it’s time to configure a new project for Jetpack Compose. This involves creating a new Android project and configuring it to leverage Compose for UI development.
- Configuring a New Project for Jetpack Compose: When starting a new Android project in Android Studio, make sure to choose a template that supports Jetpack Compose. During project setup:
- Select ‘Empty Compose Activity’: This template is pre-configured with the necessary Compose dependencies and configurations.
- Min SDK Version: Jetpack Compose supports Android devices with API level 21 and above. Ensure your
minSdkVersion
is set accordingly.
- Adding Necessary Dependencies in the build.gradle File: If you didn’t select the Compose template initially, or if you’re integrating Compose into an existing project, manually add the required dependencies. Open your app’s
build.gradle
file, add the dependencies listed above under the dependencies block, and ensure thatcomposeOptions
is enabled with the latest stable version of Jetpack Compose. - Enabling Jetpack Compose Support: Jetpack Compose needs explicit enabling in the project’s build configuration:
- Within the
android
block of your app-levelbuild.gradle
file, setbuildFeatures { compose true }
. - This flag activates Compose-specific features like composables, previews, and other Compose tooling within Android Studio.
- Within the
By following these steps, your project will be fully set up to leverage Jetpack Compose for modern Android development. Now, you’re ready to dive into creating stunning, reactive, and maintainable UIs with Jetpack Compose.
Code Example: Counter App in Jetpack Compose
Here’s a simple example of a counter app built using Jetpack Compose. This demonstrates the declarative nature of Compose, where the UI is updated based on state changes.
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.foundation.layout.*
@Composable
fun CounterApp() {
var count by remember { mutableStateOf(0) }
Column(
modifier = Modifier
.fillMaxSize()
.padding(16.dp),
verticalArrangement = Arrangement.Center
) {
Text(text = "Count: $count", style = MaterialTheme.typography.h4)
Button(onClick = { count++ }, modifier = Modifier.padding(top = 16.dp)) {
Text(text = "Increment")
}
}
}
@Preview
@Composable
fun PreviewCounterApp() {
CounterApp()
}
The Need for Jetpack Compose
Jetpack Compose addresses several pain points developers faced with the traditional View system:
- Simplification: Developers no longer need to juggle between XML layouts and Kotlin code. Compose offers a unified development model with minimal boilerplate.
- Modern Development Paradigm: The declarative nature of Jetpack Compose is aligned with modern UI development patterns used by frameworks like React and Flutter. This makes it easier for developers to build dynamic, state-driven UIs.
- Better Performance: By minimizing UI hierarchies and offering smarter recomposition strategies, Jetpack Compose improves performance, especially for complex UIs.
- Faster Development Cycles: With tools like Live Previews, Compose allows for quicker iteration, reducing the time to build and test UIs.
Recent Updates in Jetpack Compose
Jetpack Compose has been rapidly evolving with new updates aimed at making it more robust and feature-rich. Here are some key recent developments:
- Wear OS Support: Compose now supports Wear OS, making it easier to build apps for wearable devices using the same API.
- Material Design 3: Compose now offers native support for Material Design 3 components, enabling developers to create UIs aligned with the latest Google design standards.
- Animation Improvements: Recent updates include new APIs for handling animations and transitions, making it easier to build smooth and dynamic UIs.
- Interoperability with View: Google has improved the interoperability between Compose and traditional View-based layouts, allowing gradual adoption of Compose in existing projects.
Essential Tips for Jetpack Compose
- 1. Use
remember
for State Persistence: Avoid recomposition issues by usingremember
to persist state across recompositions.
var count by remember { mutableStateOf(0) }
- 2. Optimize Recomposition with
rememberUpdatedState
: UserememberUpdatedState
to maintain the latest state in callbacks without triggering recompositions unnecessarily.
val currentValue = rememberUpdatedState(newValue)
- 3. Leverage
LazyColumn
andLazyRow
for Large Lists: Instead of usingColumn
orRow
, which renders all items at once, useLazyColumn
orLazyRow
to render only visible items, improving performance.
LazyColumn {
items(itemsList) { item ->
Text(text = item)
}
}
- 4. Master Modifiers for Layout and Styling: Modifiers control the layout, styling, and behavior of your UI components. Apply modifiers in the correct order to ensure the desired layout.
Text(
text = "Hello, World!",
modifier = Modifier
.padding(16.dp)
.fillMaxWidth()
.align(Alignment.CenterHorizontally)
)
- 5. Handle State Hoisting: For reusability, manage state higher up in the composable tree and pass it down to child composables.
@Composable
fun ParentComposable() {
var count by remember { mutableStateOf(0) }
ChildComposable(count = count, onIncrement = { count++ })
}
@Composable
fun ChildComposable(count: Int, onIncrement: () -> Unit) {
Text(text = "Count: $count")
Button(onClick = onIncrement) {
Text("Increment")
}
}
Conclusion
In conclusion, Jetpack Compose revolutionizes Android development, offering a modern, efficient way to build stunning UIs. As you embark on mastering this tool, your apps will not only look better but also perform seamlessly. Ready to elevate your Android development game? Contact Bluetick Consultants today to see how we can help you integrate Jetpack Compose into your projects!